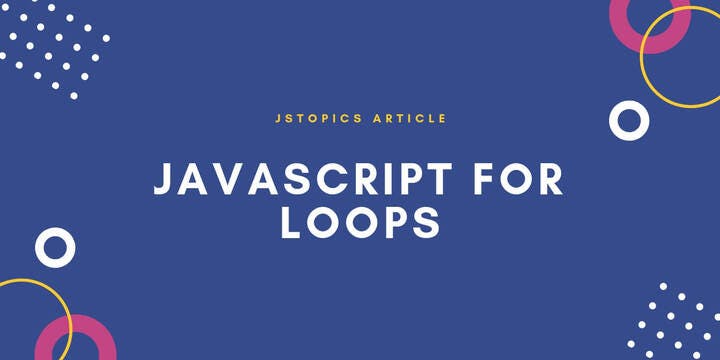
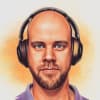
JavaScript *for* Loops
For loop is one mechanism used everywhere in JavaScript. But are you using this loop efficiently?
JavaScript for loops
Loops are incredibly useful “tools” in programming! Since we as a programmers work with collections, arrays, lists of data, we need to iterate over all the time to manipulate or mutate or console.log();
😊 And there are many different kinds of loops, especially across the whole landscape of programming languages. Loop keywords, vary from your usual while, switch
etc. to as ridiculous as the languages that use them: STICK AROUND(ArnoldC), IM IN YR LOOP(LOLCODE). But one loop that stands out, is the mighty for loop.
Why Use For Loop in JavaScript?
Well, as already stated, for
loop is very useful in programming. In JavaScript (and probably in most languages) it is the most used kind of loop. JavaScript for loop is widely used in great libraries like Underscore.js
. Alright, enough talk, let’s have a look at our basic JavaScript for loop.
for (let i = 0; i < 5; i++) {
console.log('We are in iteration: ' + i);
}
First line is where we do all of our for loop setup. It consists of three parts (expressions) divided by semicolon. All of these parts are optional.
let i = 0
First, we define starting value of index, and initial loop variables. We want our loop to start from 0, so we set our index variable i to 0.
i < 5
Second part is the actual condition, which evaluates to boolean, that is checked before each loop iteration is run. Beware it is very easy to create infinite loop here, if our condition always evaluates to true, we have infinite loop.
i++
And finally, the third part of the setup is our final-expression. Here we usually update our index, as we can see in the code example (we are incrementing our index value). This part runs before the next loop iteration.
Now let's have a look at some JavaScript for loop tips.
Tip 1: Cache the Array Length
What do I mean by caching the length? It's simply just setting the length of the array we want to iterate over to a variable. The point is, we don’t want to ask for it every loop iteration because it is more performance intensive than asking for variable value. Here is an example:
let myArray = []
for (let i = 0; i < 10000; i++) {
myArray.push(i)
}
console.time("loop test no cache");
for (let i = 0; i < myArray.length; i++) {
let j = i;
}
console.timeEnd("loop test no cache");
console.time("loop test with cache");
for (let i = 0, arrayLength = myArray.length; i < arrayLength; i++) {
let j = i;
}
console.timeEnd("loop test with cache");
I have added console.time
to check the performance and on my machine the difference is quite big, even though it might not seem.
node app.js
loop test no cache: 0.16ms
loop test with cache: 0.074ms
Of course, this is a very simple example and there is a lot of inputs that go into actual overall performance. But if we were working with some HTML DOM arrays, and would be asking for their length at each iteration, the difference in performance would be even greater. Good rule of thumb is, when you don’t need dynamic length of the array in your for loop, always cache the array length.
Tip 2: JavaScript for loop Variable Name
This tip is very subjective but I like it and it might help some of you. It has got to do with how we name our variable that acts as index in the for loop. It’s best explained on code example.
for (let ii = 0; ii < 2; ii += 1) {
for (let iii = 0; iii < 5; iii += 1) {
console.log('ii is now: ' + ii + ' and iii is now: ' + iii);
}
}
As we can see, I am using ii for the name of my index. Why? Well, once in a while I come across the bug within the loop so I want to find it. If I would name the variable i
and would search for i
in VSCode, I would get gazillion results. This way, when I search for ii
, I find it right away. I know I could change my search query to something like let i = or similar. There is many ways to do everything in programming and that is awesome about it. I am not saying this is JavaScript best practice, I’m just saying, maybe give it a try, if you like it stick with it, otherwise let’s go to the final tip.
Tip 3: Comparing To Zero
This one is more of a micro-optimisation. In JavaScript, it is usually faster (not by much) to compare to 0 than to anything else like the length of the array. So let’s have a look on how to do this small performance optimisation.
let myArr = [1,2,3];
console.time("loop test compare to number");
for (let i = 1, max = myArr.length; i < max; i++) {
let j = i;
}
console.timeEnd("loop test compare to number");
console.time("loop test compare to zero");
for (let i = myArr.length; i--;) {
let j = i;
}
console.timeEnd("loop test compare to zero");
In the second for loop we assign i
to the length of the array and than just subtract from that number. The point is, condition evaluates those actual numbers. And in JavaScript, all of the numbers besides 0, return as a true boolean value. So when the i will equal to 0 loop will end. Great stuff!
Here are the times, almost 10x improvement?
node app.js
loop test compare to number: 0.038ms
loop test compare to zero: 0.003ms
Developer Comfort Comes First
Always keep this in mind, you have to feel good using these optimisations and implementing these practices. For example, the last optimisation (Tip 3) is very very subtle, so if that loop setup doesn’t feel right for you, just don’t use it. Yes when you need to save every micro-second, than you need to consider all of the improvements possible. But 90% of the time our code won’t be needing all of the performance optimisations in the world. My rule is, I never use them at the expense of me being confused with my own code.
Developer Comfort Comes First!
That’s it for this article, thank you for reading.
Keep learning and see you in the next one!